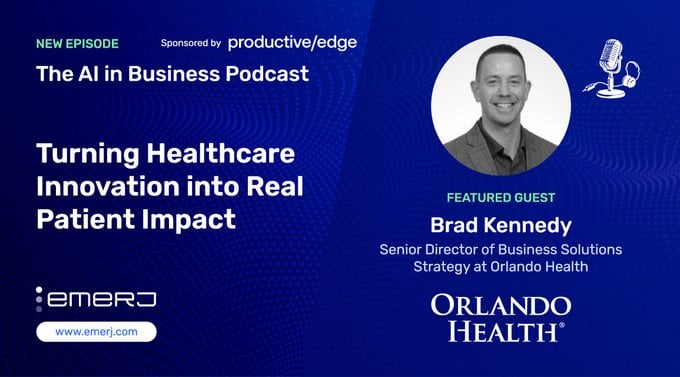
Turning Innovation into Impact: How Orlando Health Is Making AI Work for Patients
At Productive Edge, we believe healthcare innovation only matters if it delivers measurable outcomes for patients, providers, and the system as a whole. That’s exactly what Brad Kennedy from Orlando Health is focused on. In this episode of...
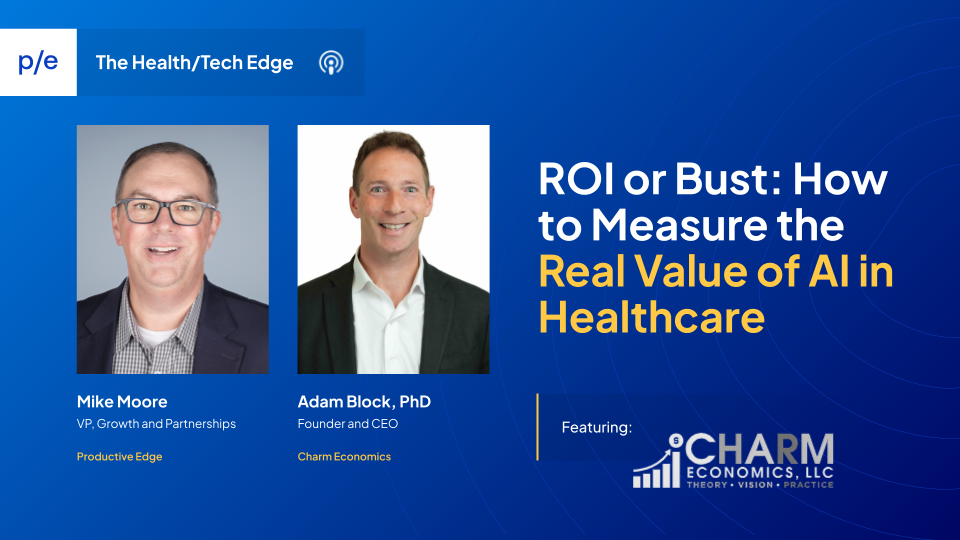
ROI or Bust: How to Measure the Real Value of AI in Healthcare
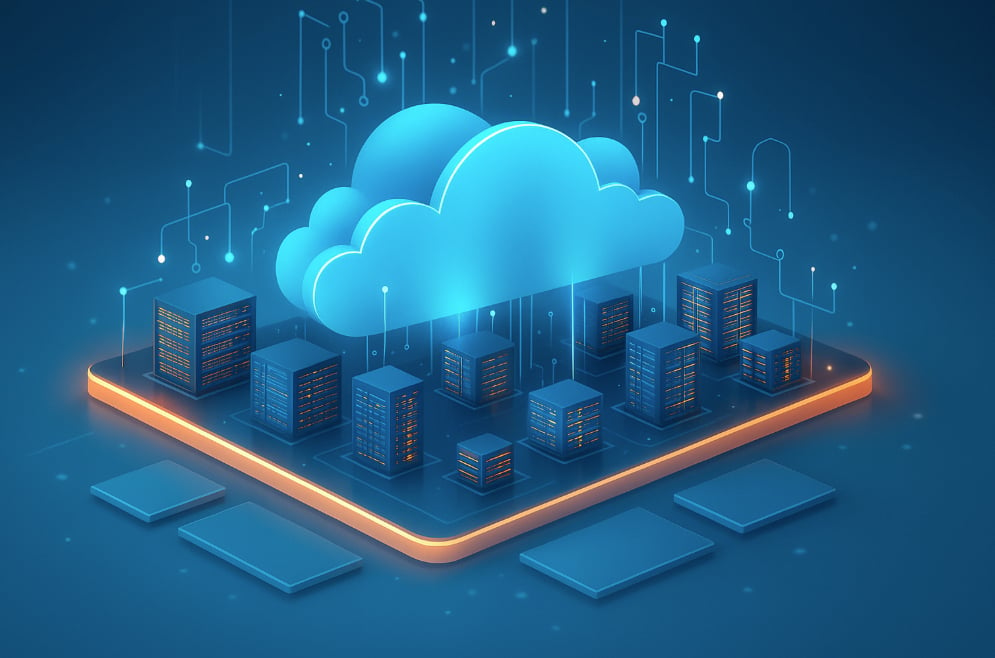
Azure Data Lake Best Practices for Healthcare Leaders
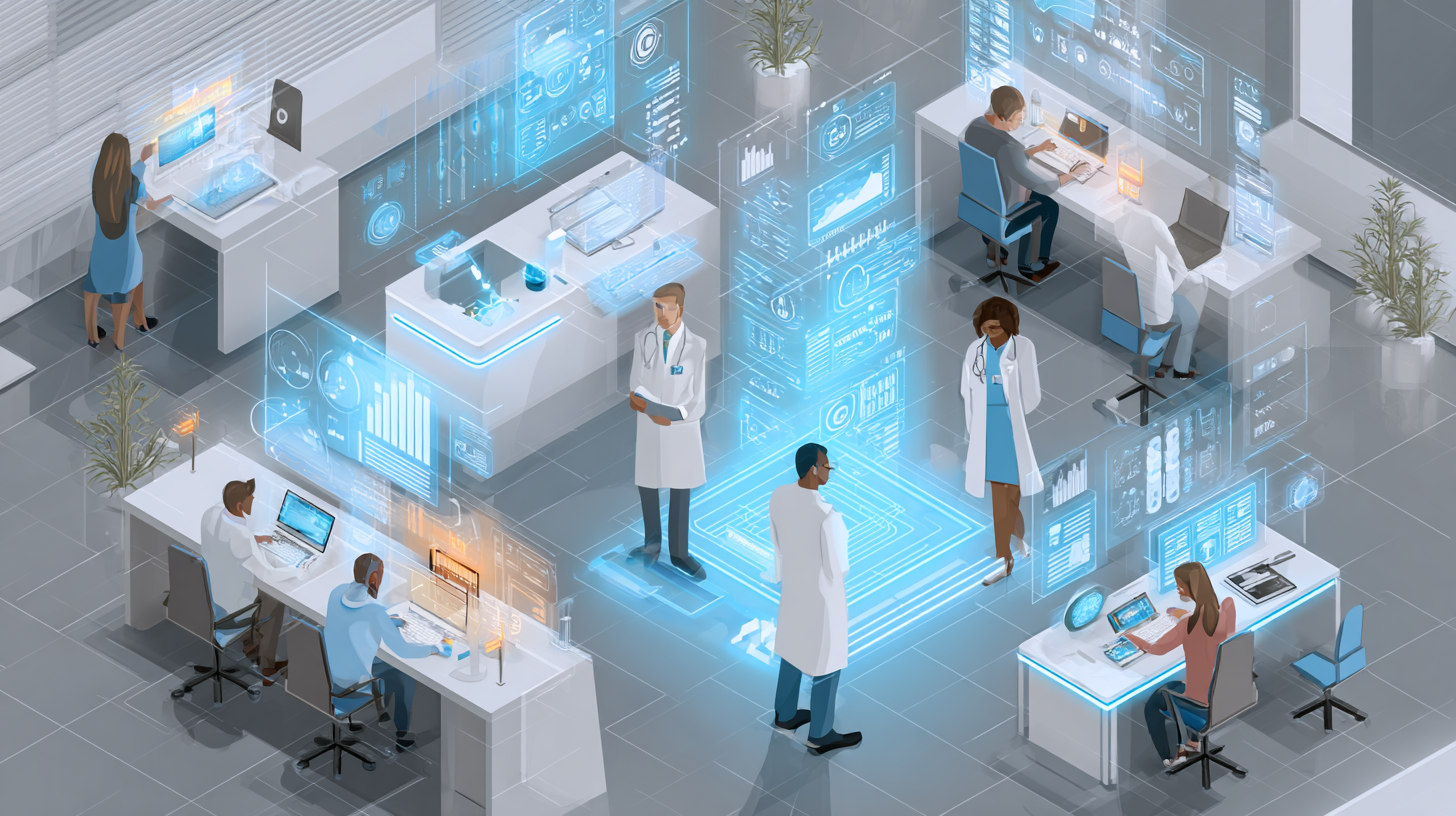
How Agentic AI is Changing Care Management
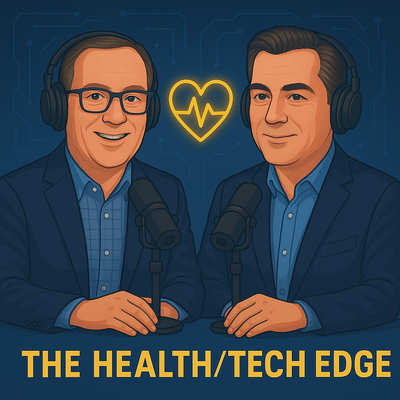
Podcast / AI Task Management
The Secret to Unlocking AI in Healthcare? Smarter Task Management
Watch Video
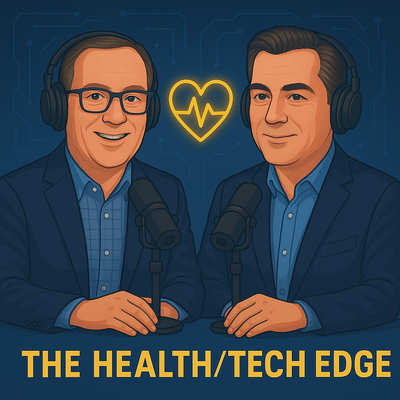
Podcast / AI & Data
From Data to Action: Building Healthcare AI Agents with Snowflake
Watch Video
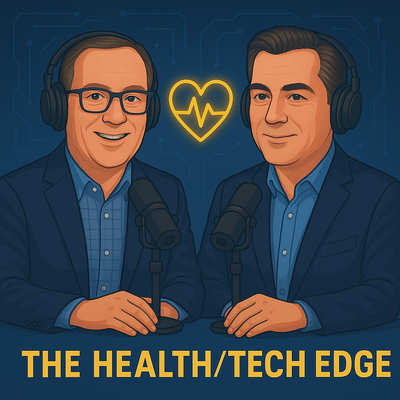
Podcast / AI Governance
Governance, Compliance, and Risk for Healthcare AI Agents
Watch Video
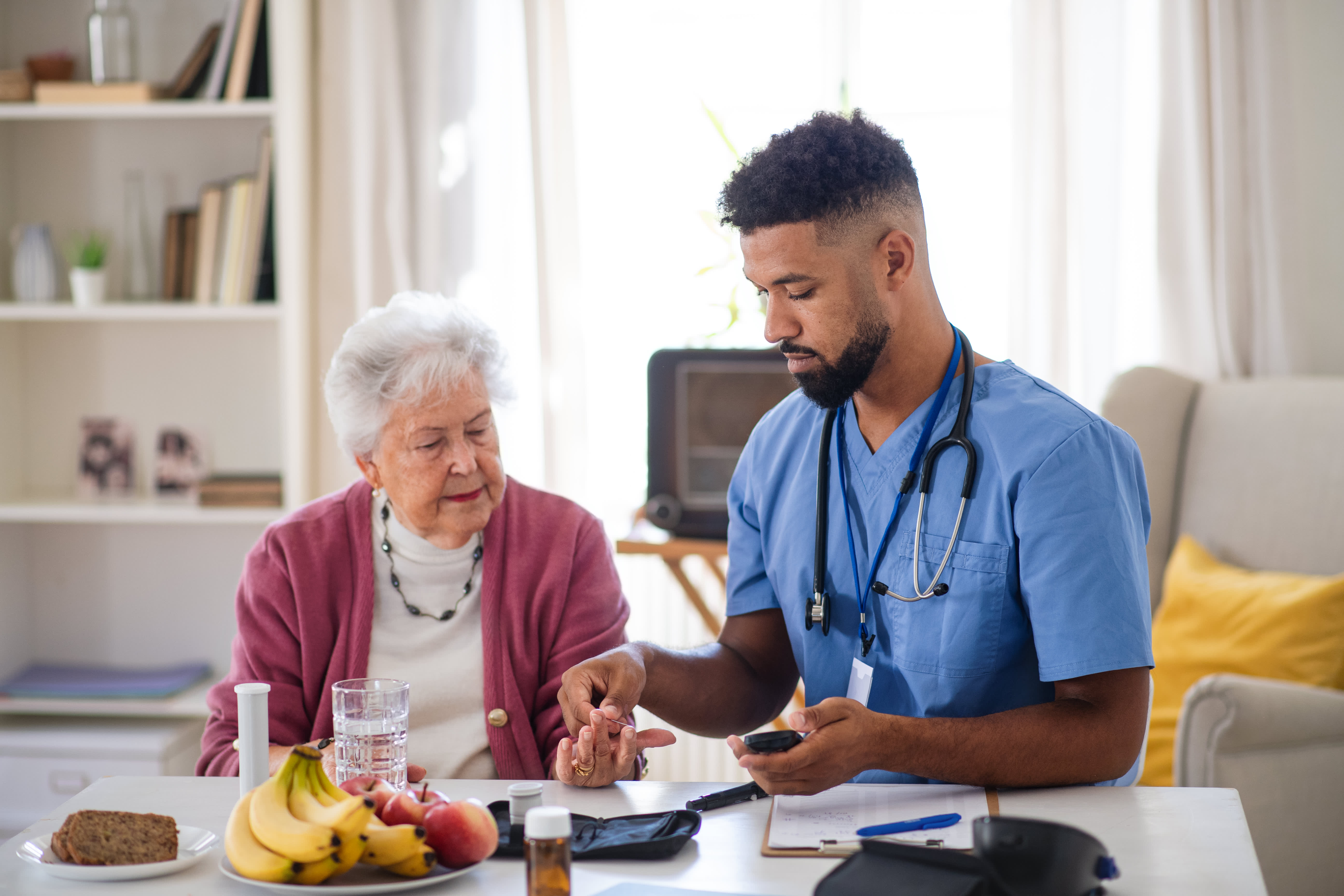
May 2025 CMS Policy Updates: What Payers and Providers Need to Know
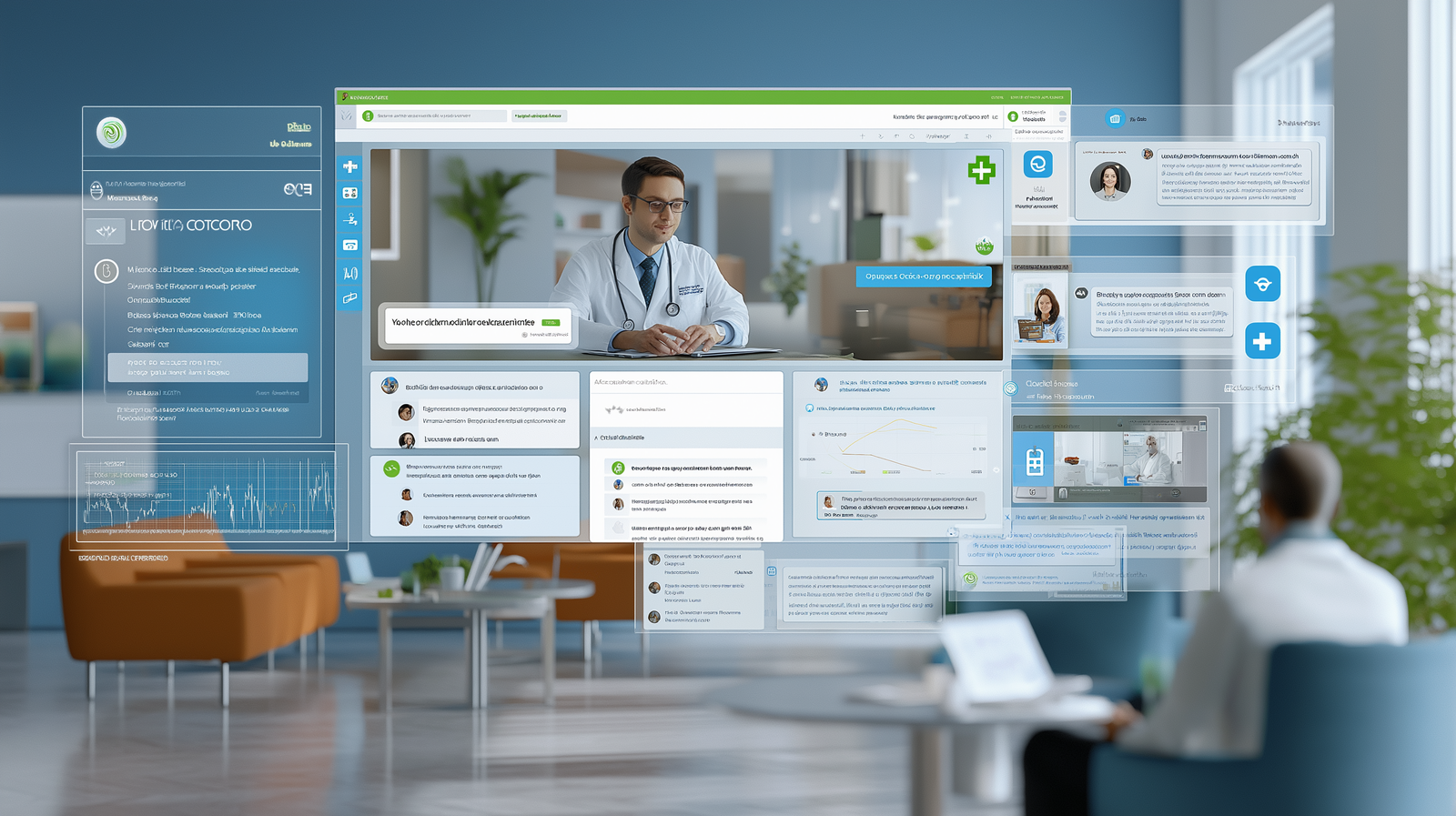
Fixing Prior Authorization: What the New CMS and HHS Pledge Means
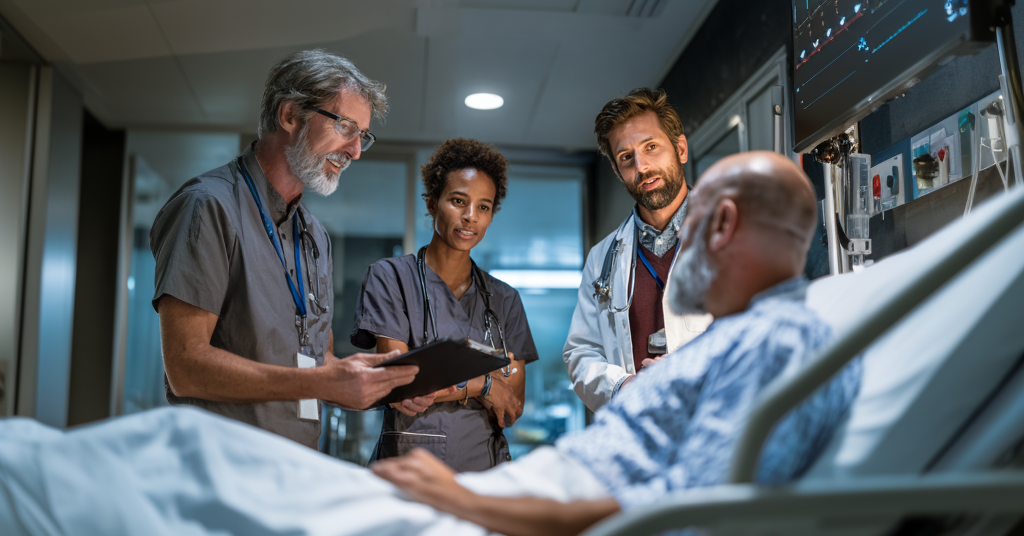
Using the Quintuple Aim to Shape an Effective AI Strategy in Healthcare
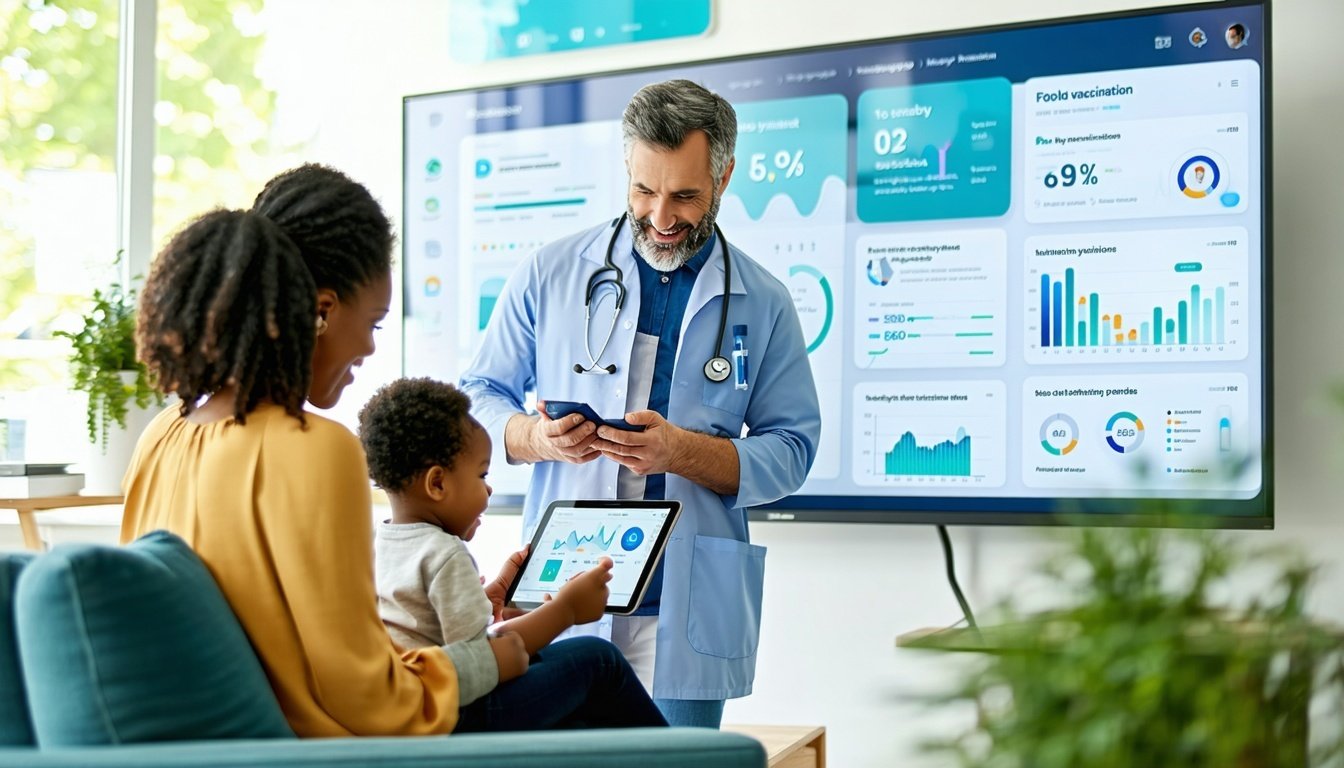
A Welcome Push Forward on Health Engagement
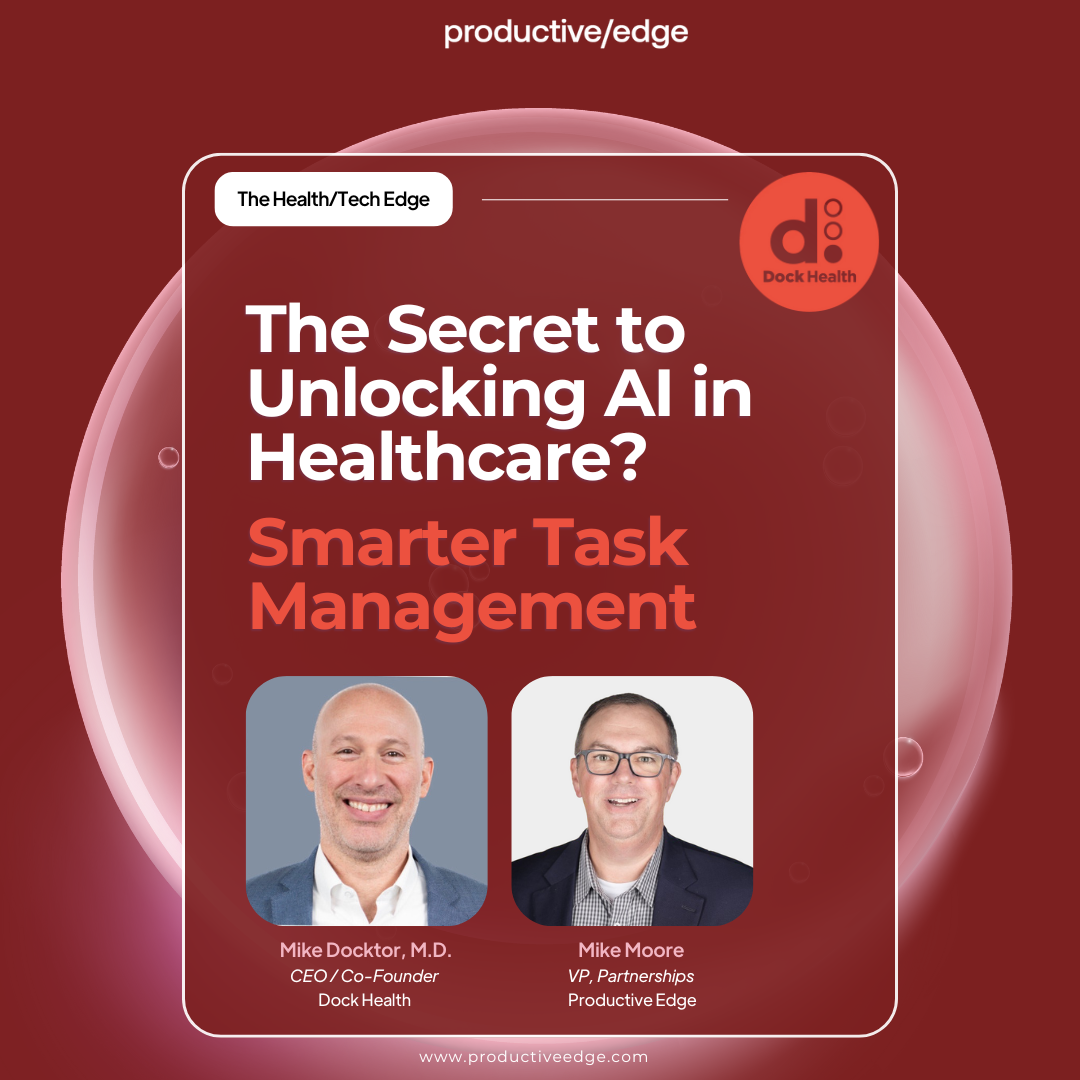
Unlocking AI in Healthcare with Smarter Task Management
