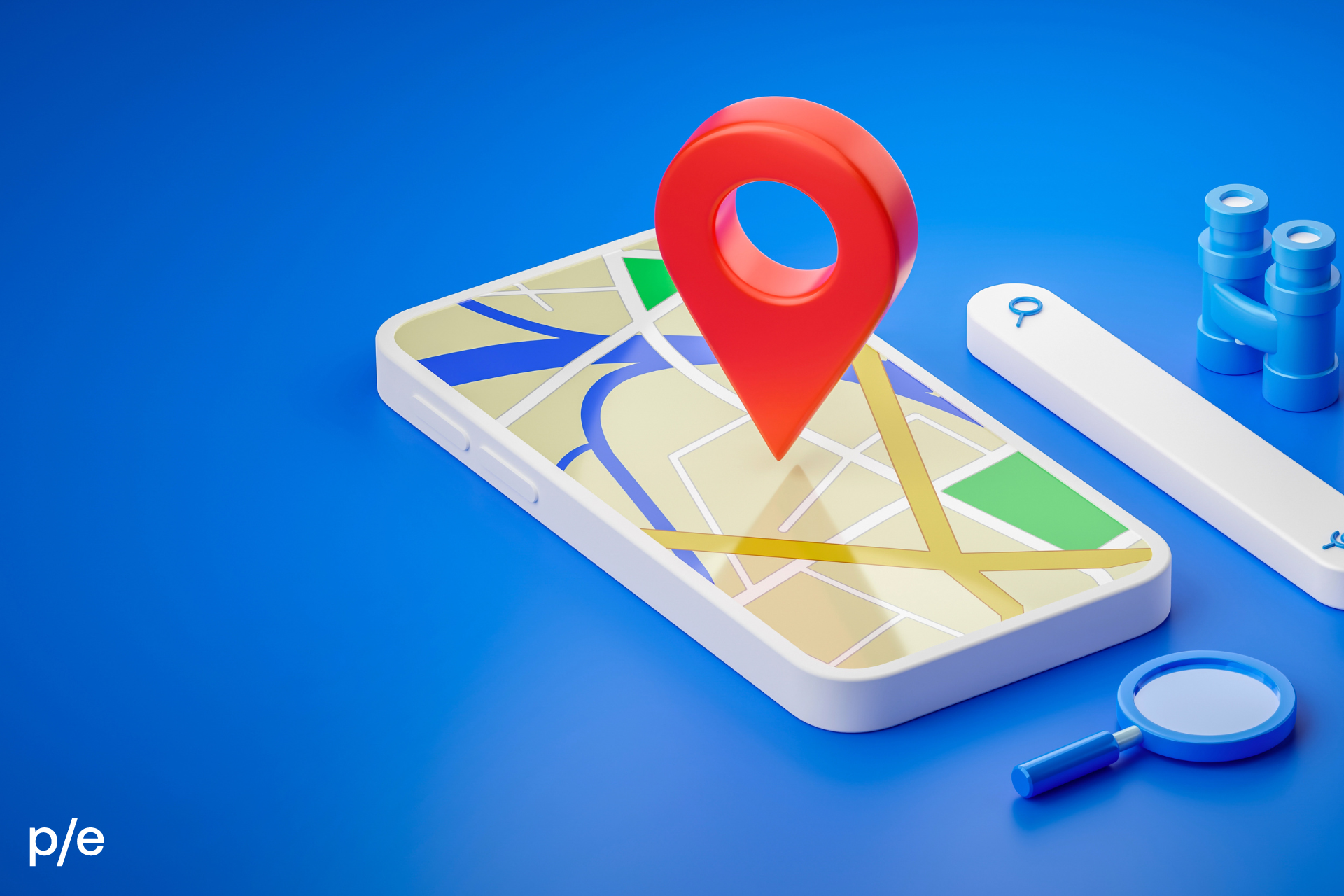
Reshaping Healthcare With Real-Time Location Solutions From CenTrak
Real-time location systems (RTLS) are building healthier, more efficient hospitals. But how do they work in real healthcare settings and what results do they enable? Find out in our recent episode of The Health/Tech Edge, a podcast...
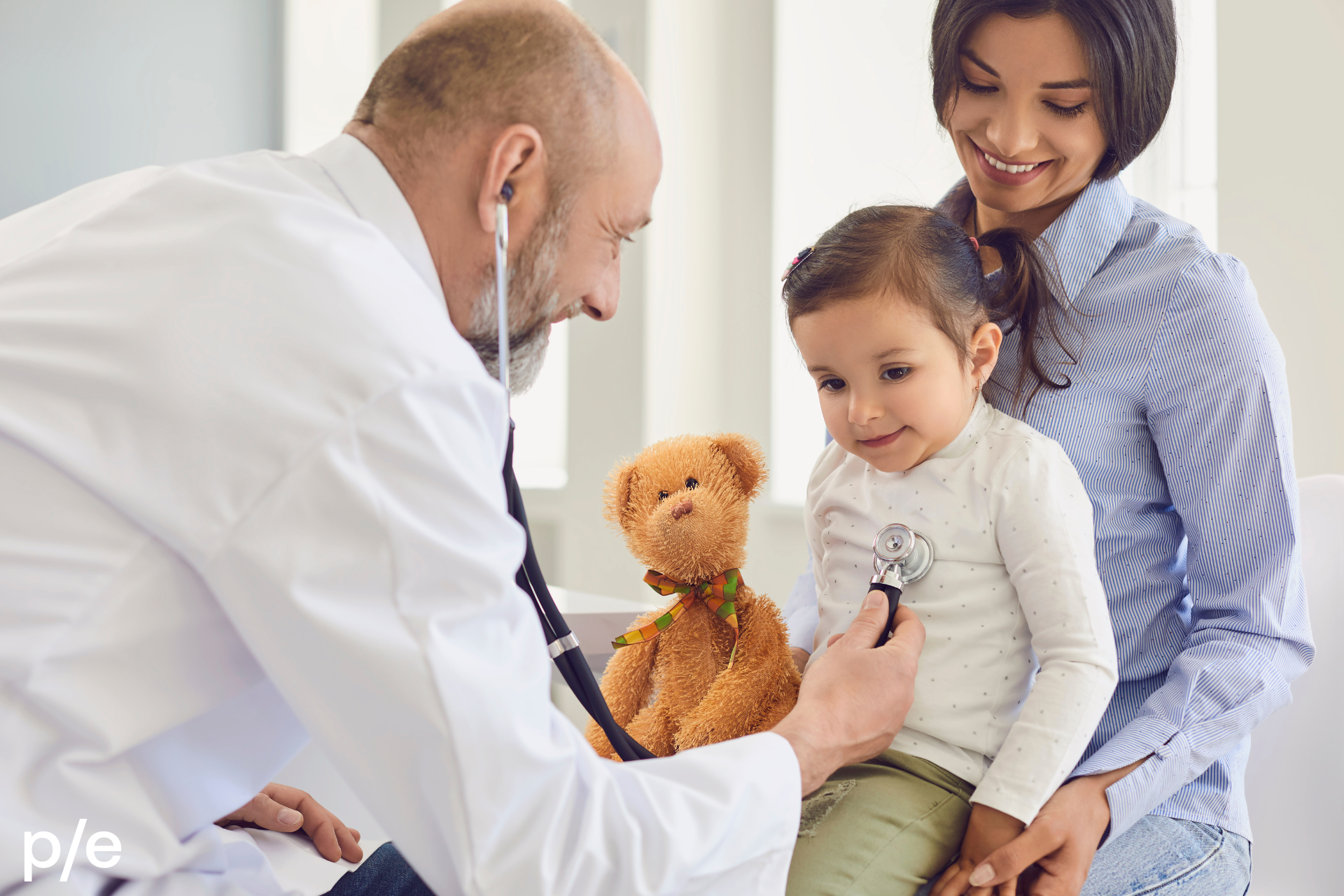
Breaking Down AI: Real Applications in Healthcare
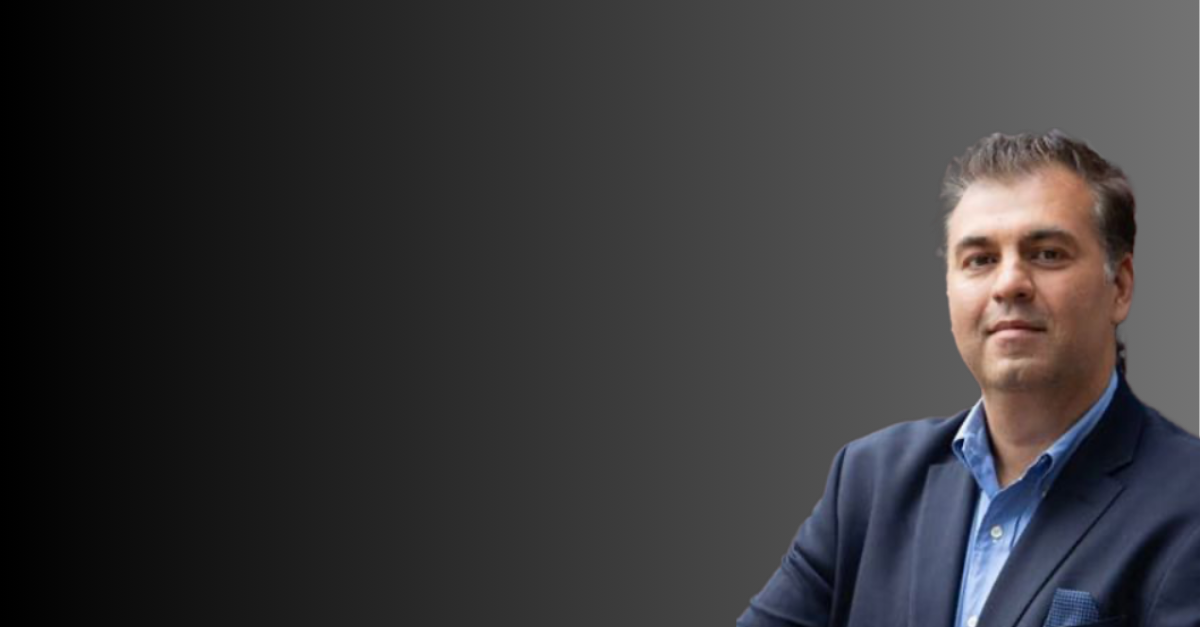
Unveiling Healthcare's Future with AI: A conversation with Becker's Healthcare
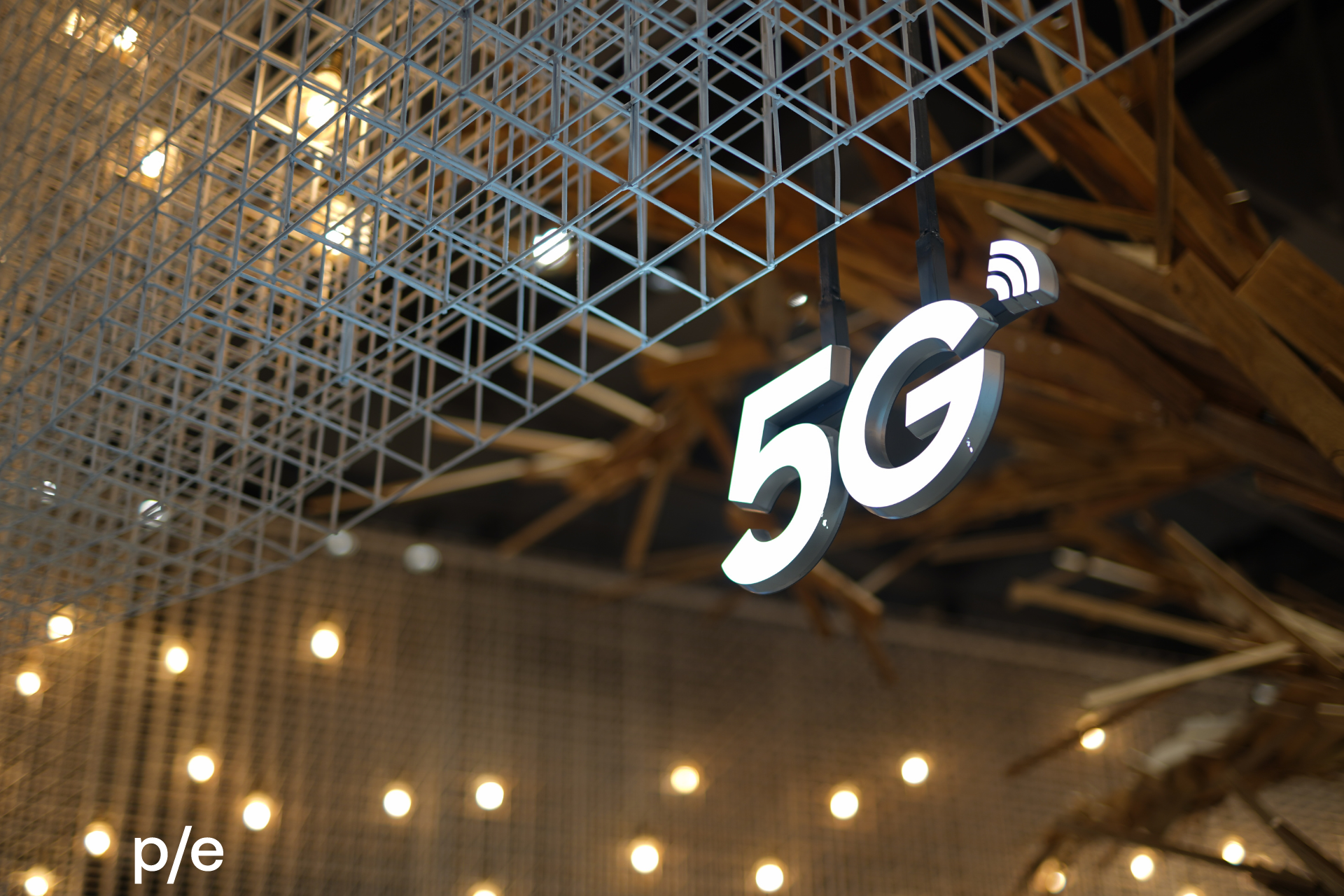
How 5G is Transforming Patient Care and Operations
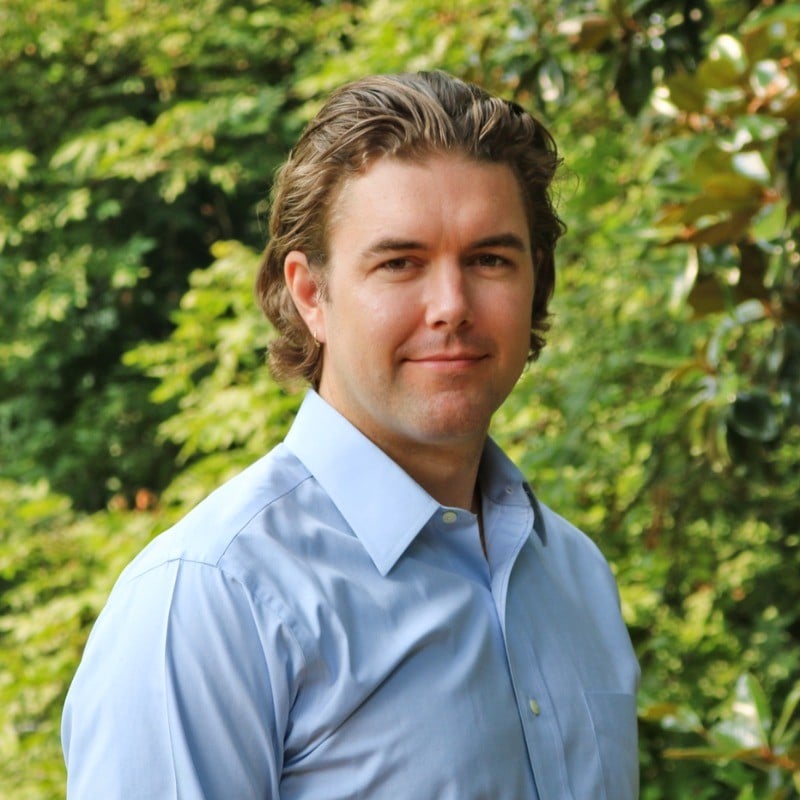
PARTNER / REDOX
Up in the Cloud: Future-Proofing Healthcare Data with Cal Harris
Watch Video
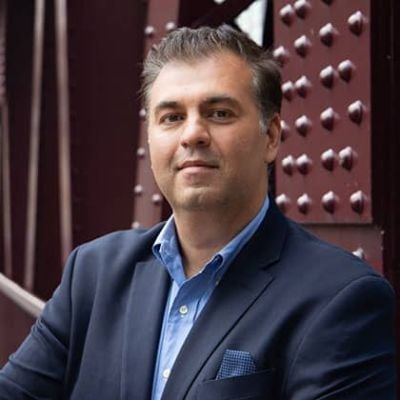
Season 2 / Episode 2
Generative AI in Healthcare: Risks & Rewards | A Deep Dive with Raheel Retiwalla
Watch Video
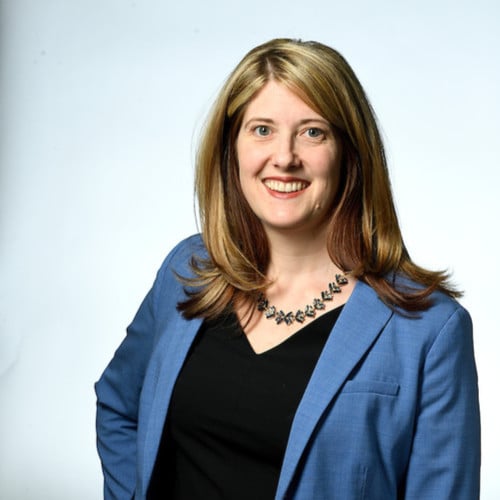
Season 2 / Episode 1
The Johns Hopkins ACG® System And Its Impact On Population Health Management with Sarah Kachur
Watch Video
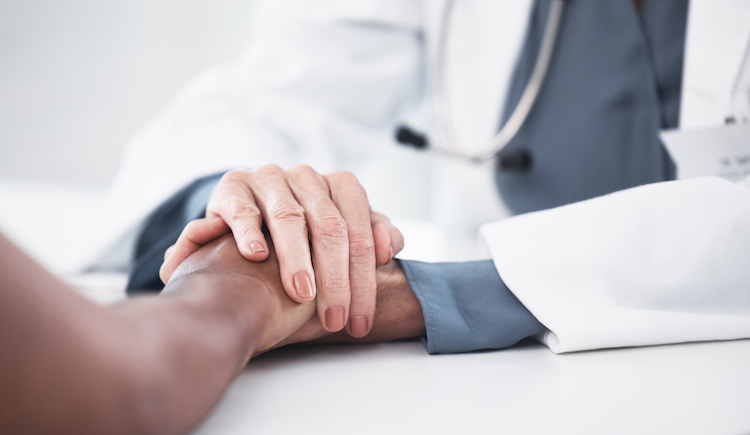
Data as an Asset to Drive Patient-Centricity and Operational Excellence
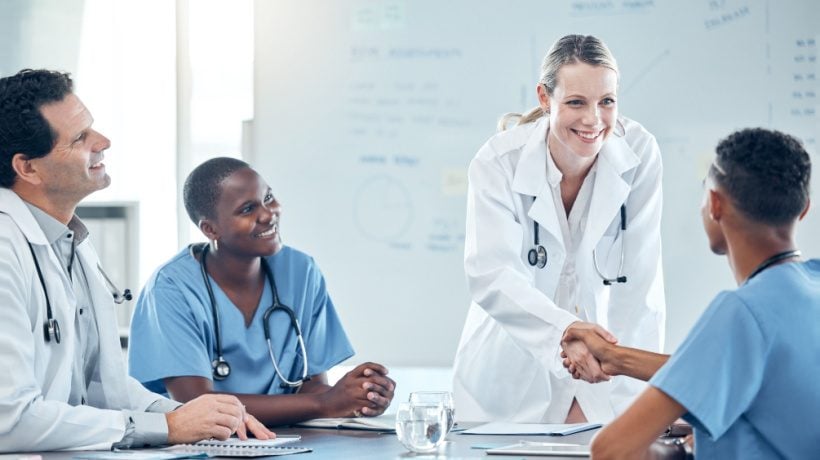
Overcoming Barriers in Healthcare Digital Transformations
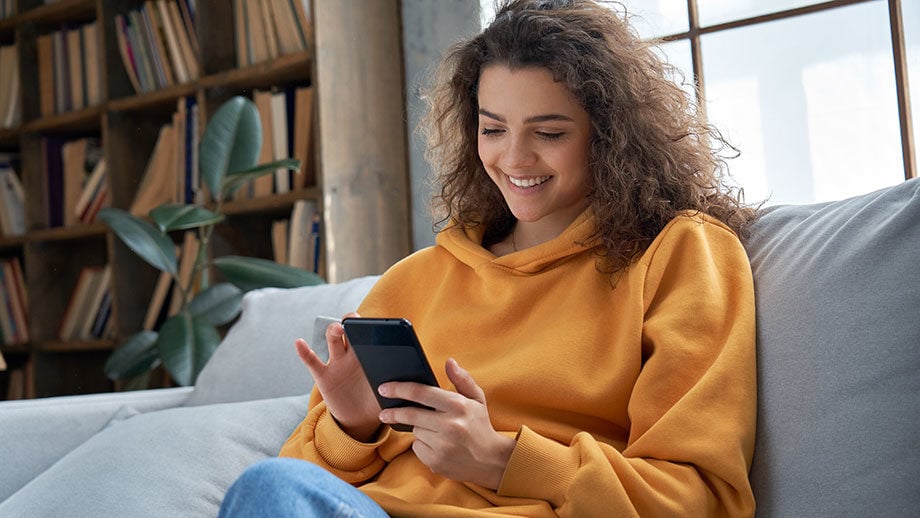
From Email To Omnichannel: Transforming Campaigns Into Scalable Digital Marketing Strategies For Enhanced Engagement
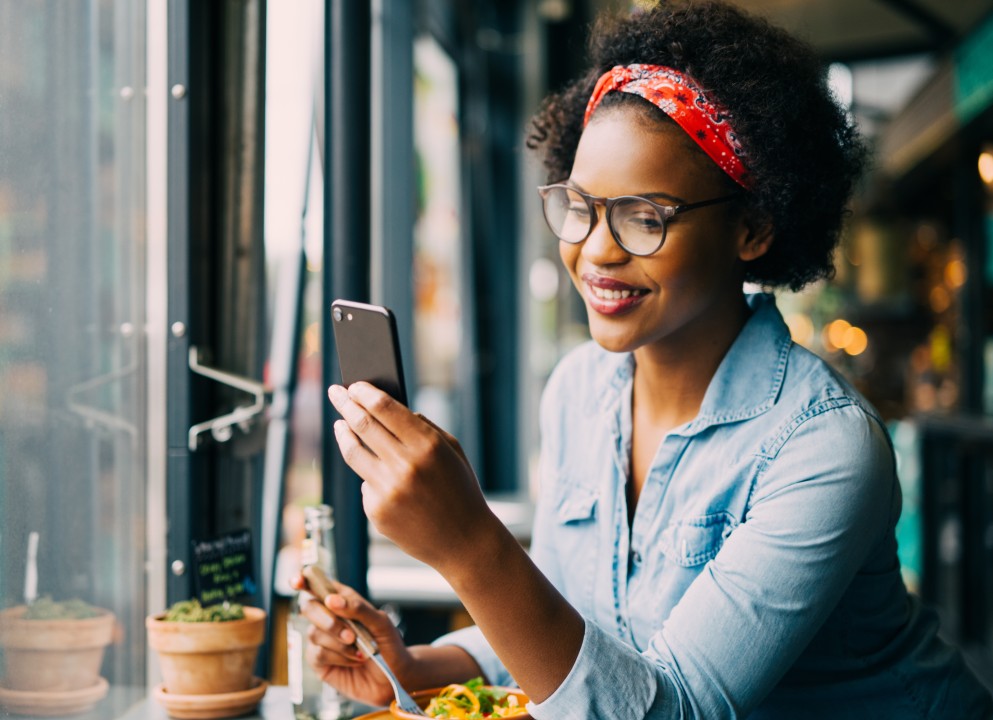
Harnessing Data to Drive Patient-Centricity and Operational Excellence
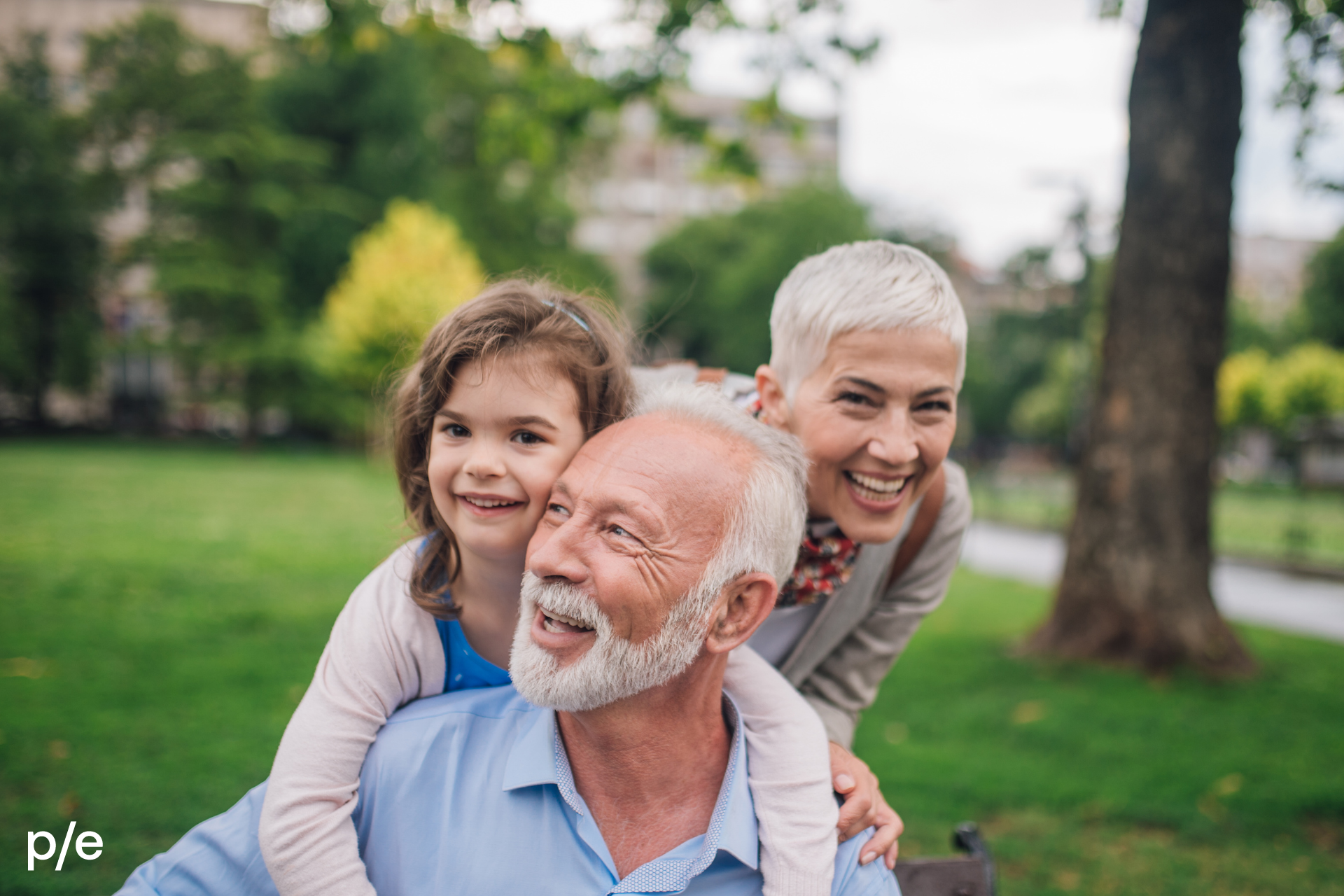
How Technology is Enabling Aging in Place
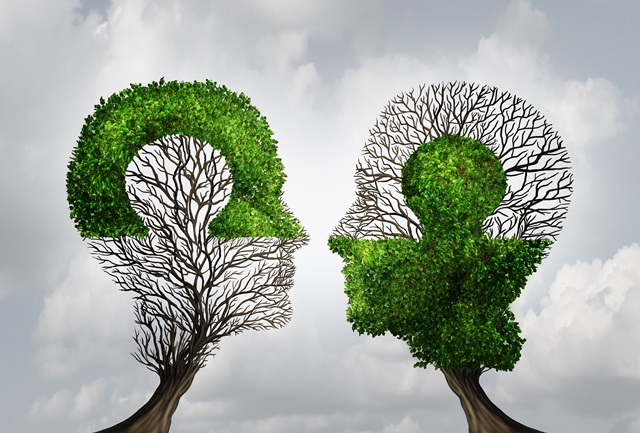