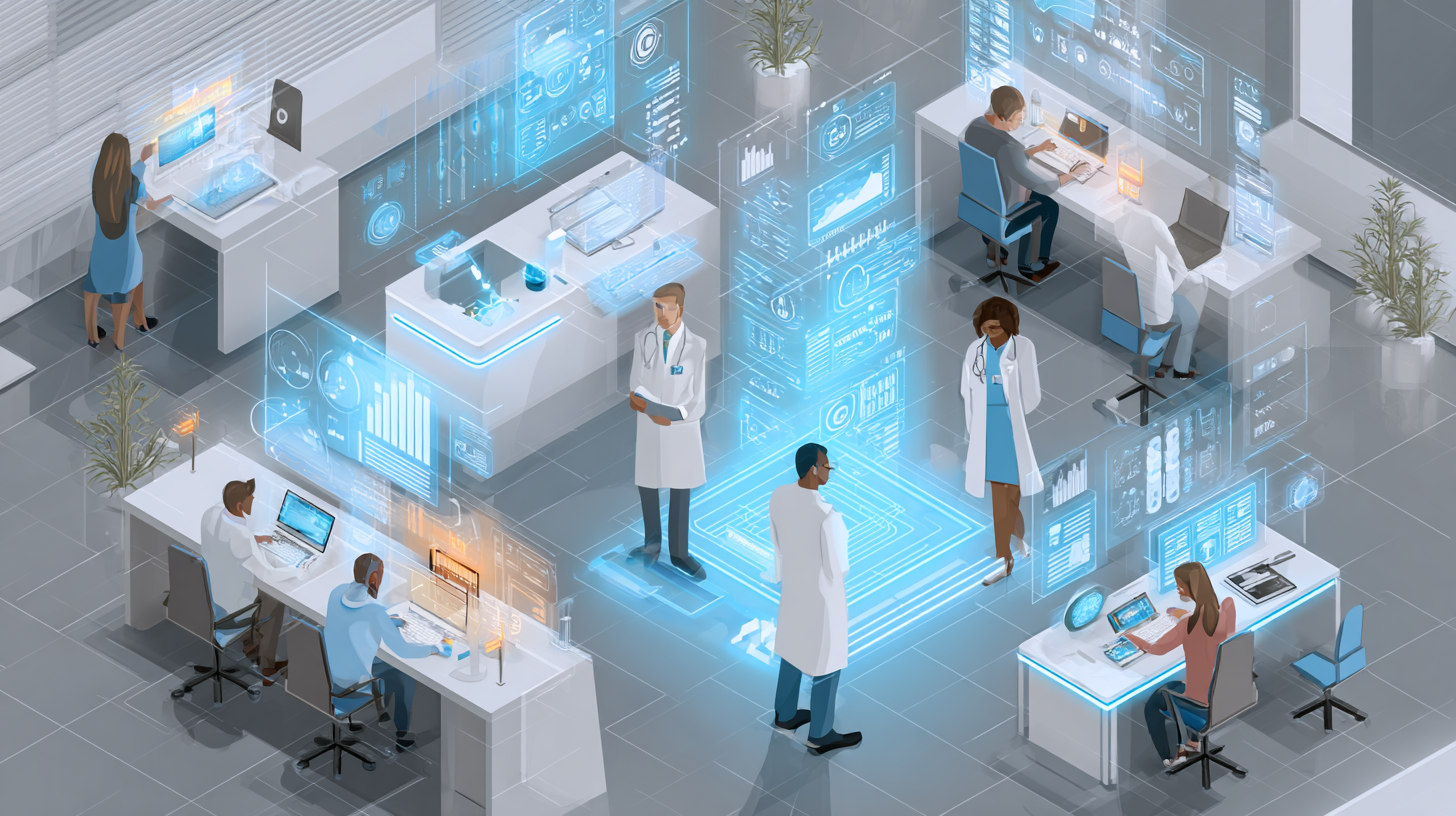
How Agentic AI is Changing Care Management
Healthcare leaders are under increasing pressure. Staffing shortages persist, chronic conditions are becoming increasingly complex, and data remains scattered across disparate systems. At the same time, value-based care models are gaining...
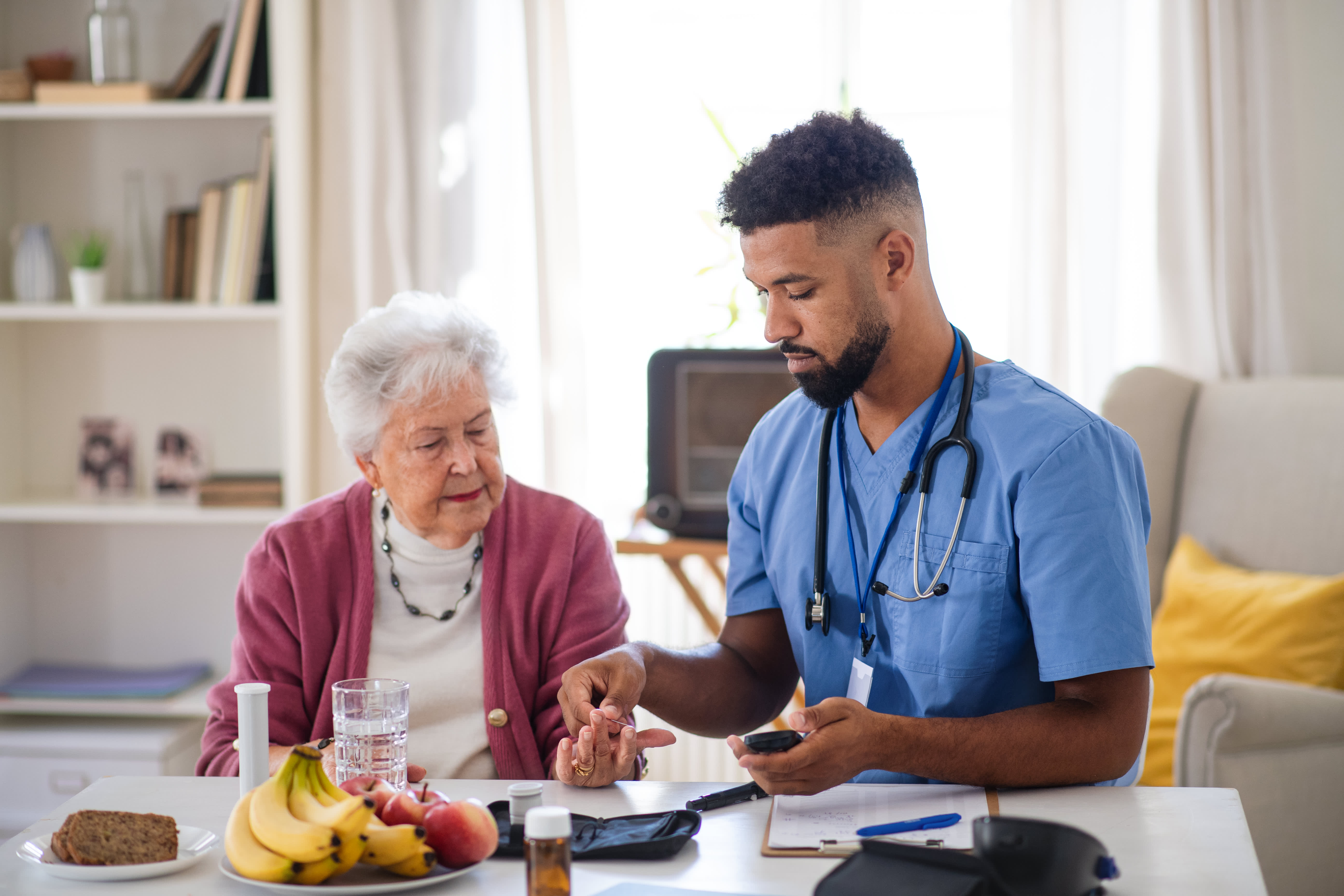
May 2025 CMS Policy Updates: What Payers and Providers Need to Know
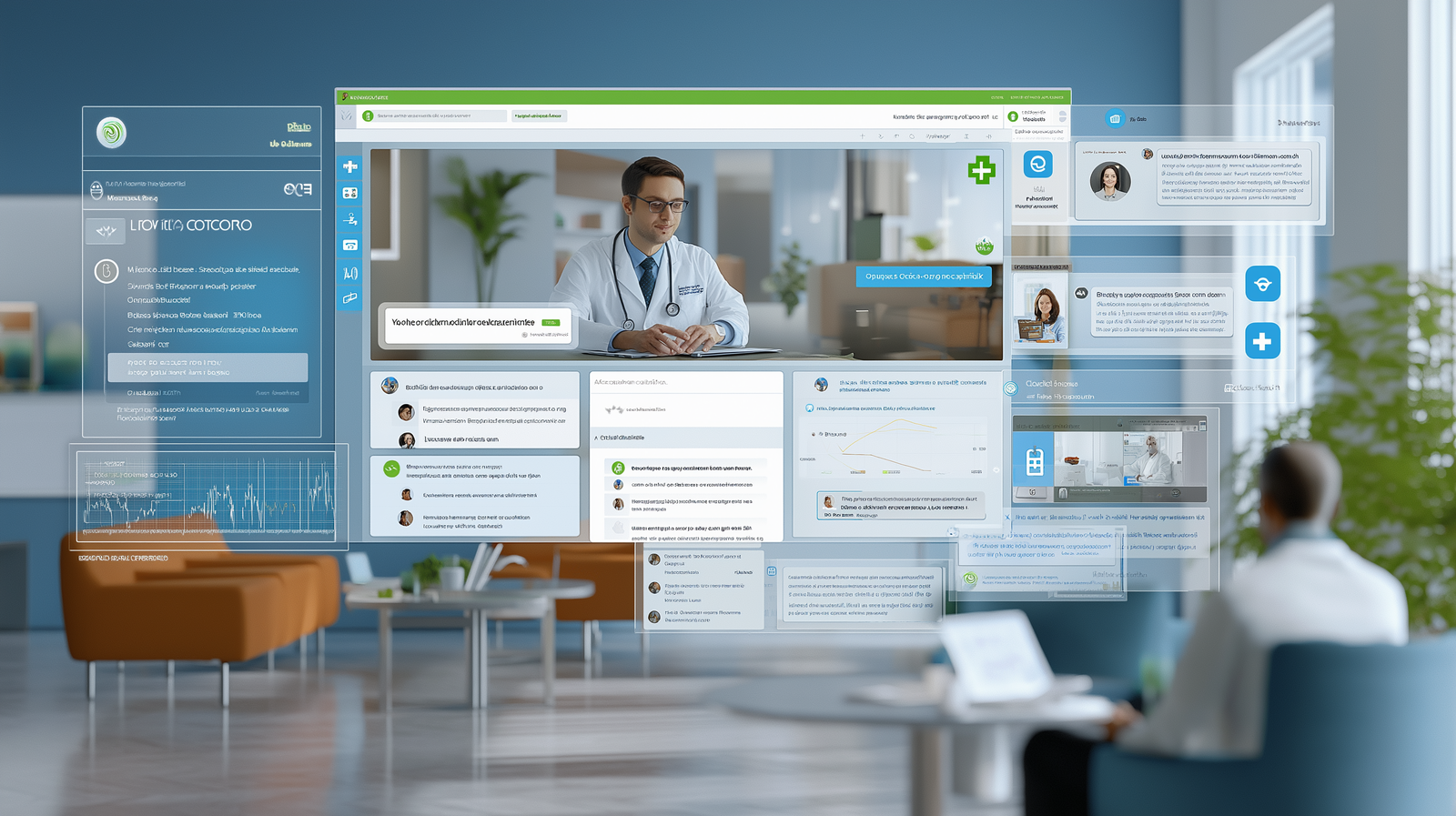
Fixing Prior Authorization: What the New CMS and HHS Pledge Means
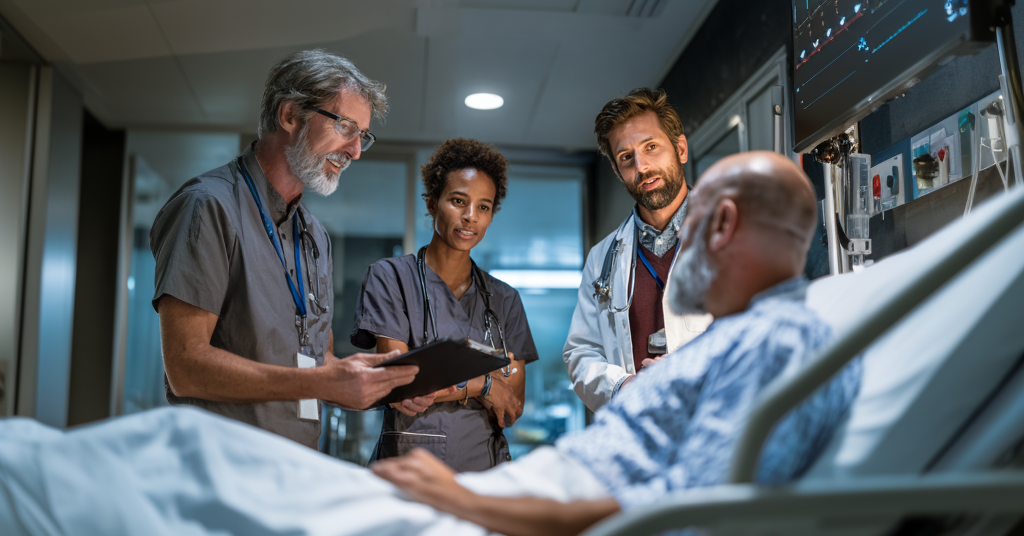
Using the Quintuple Aim to Shape an Effective AI Strategy in Healthcare
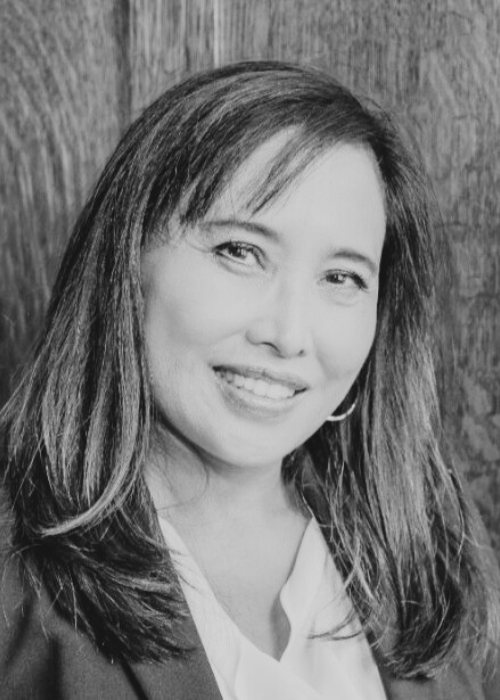
Season 2 / SITECORE
The changing role of the CMO in healthcare
Watch Video
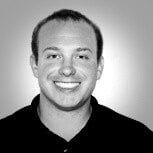
Season 2 / CENTRAK
Realtime location solutions for healthcare
Watch Video
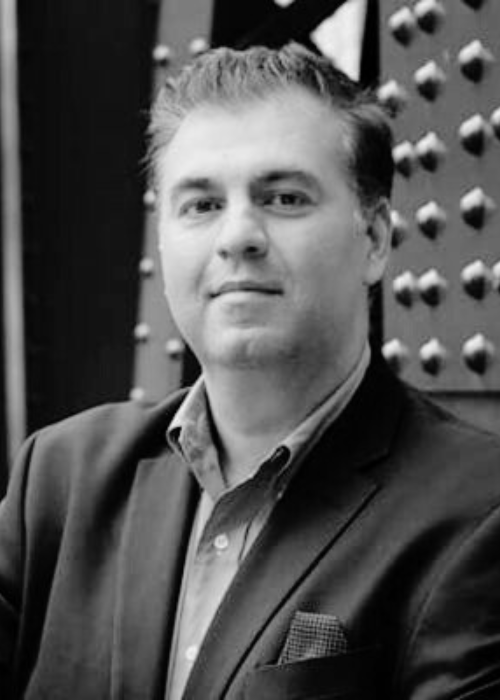
Season 2 / PE
Generative AI in healthcare: risks and rewards
Watch Video
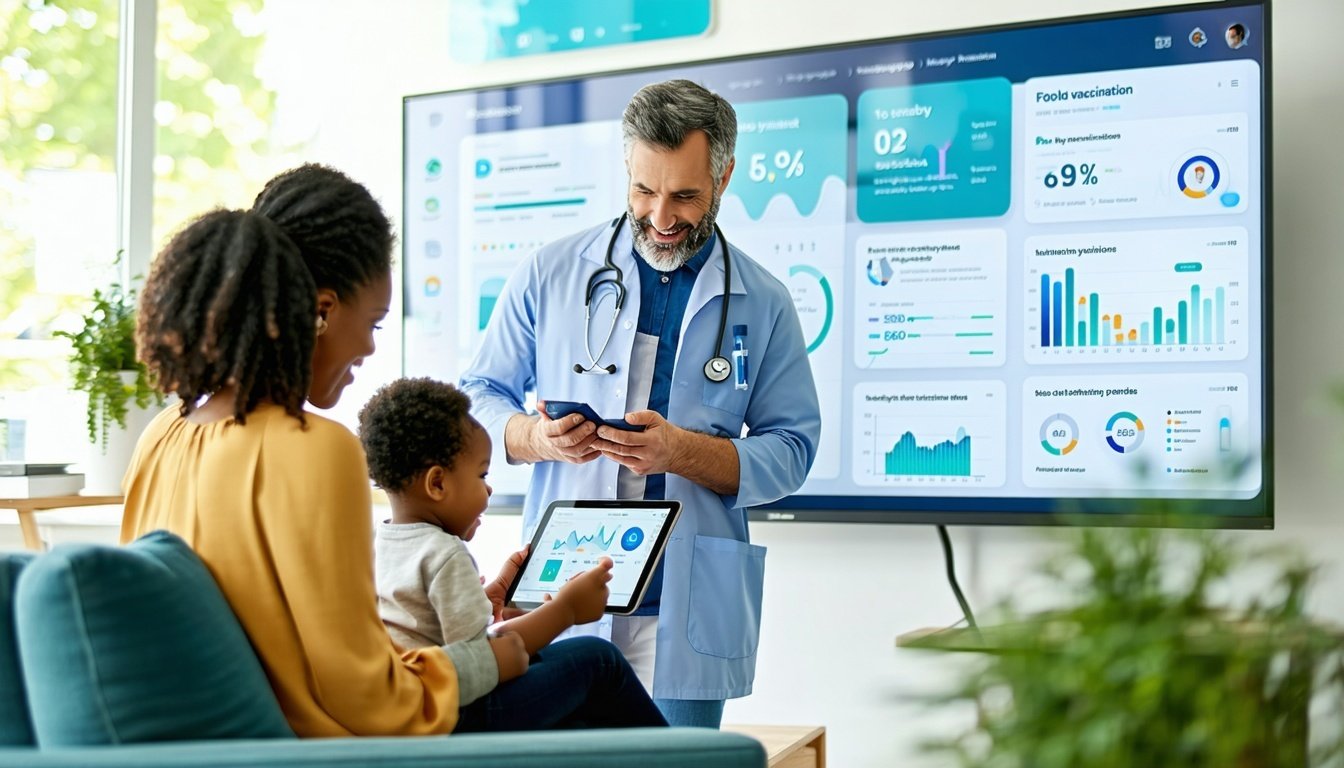
A Welcome Push Forward on Health Engagement
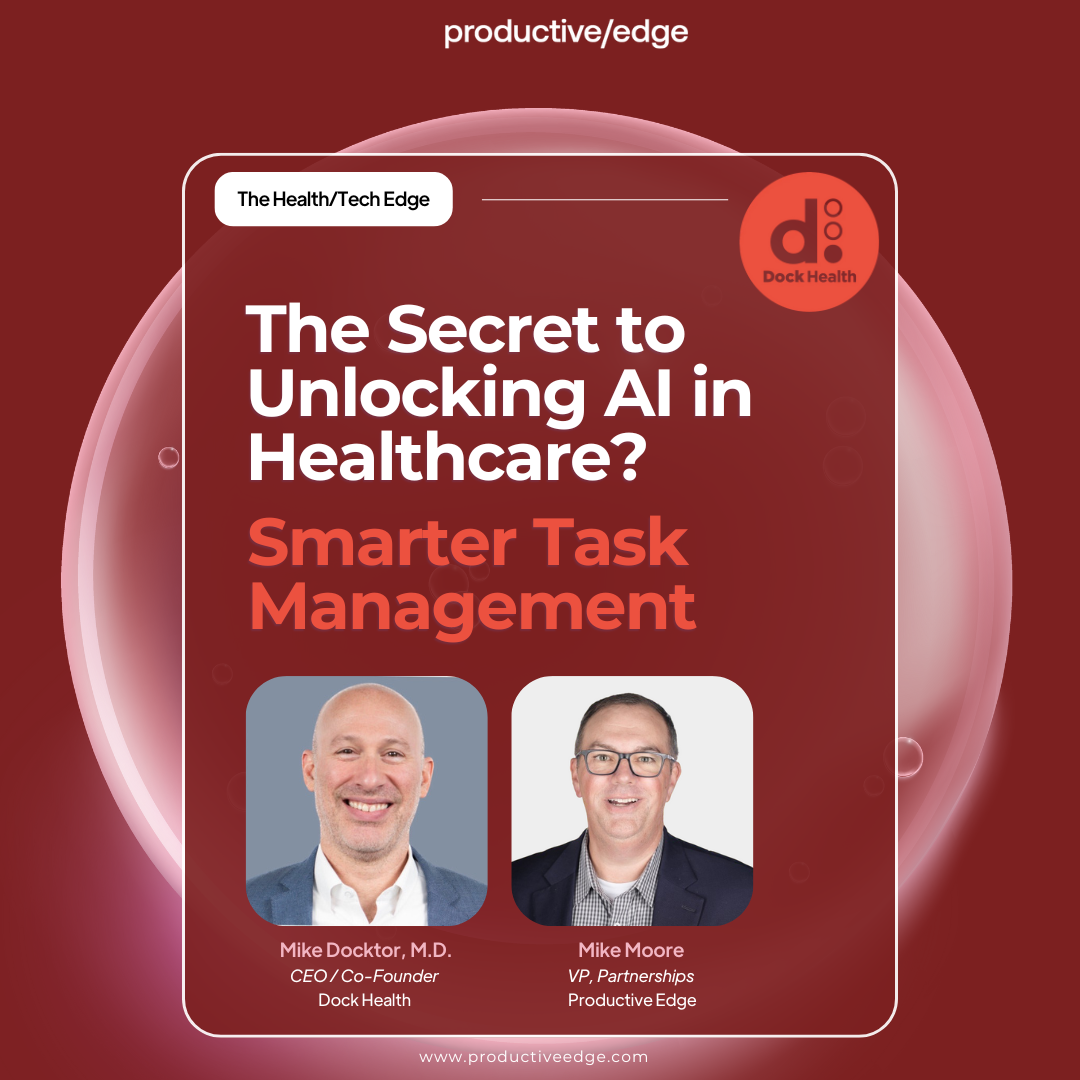
Unlocking AI in Healthcare with Smarter Task Management

How AI Agents Are Unlocking 24/7 Patient Support
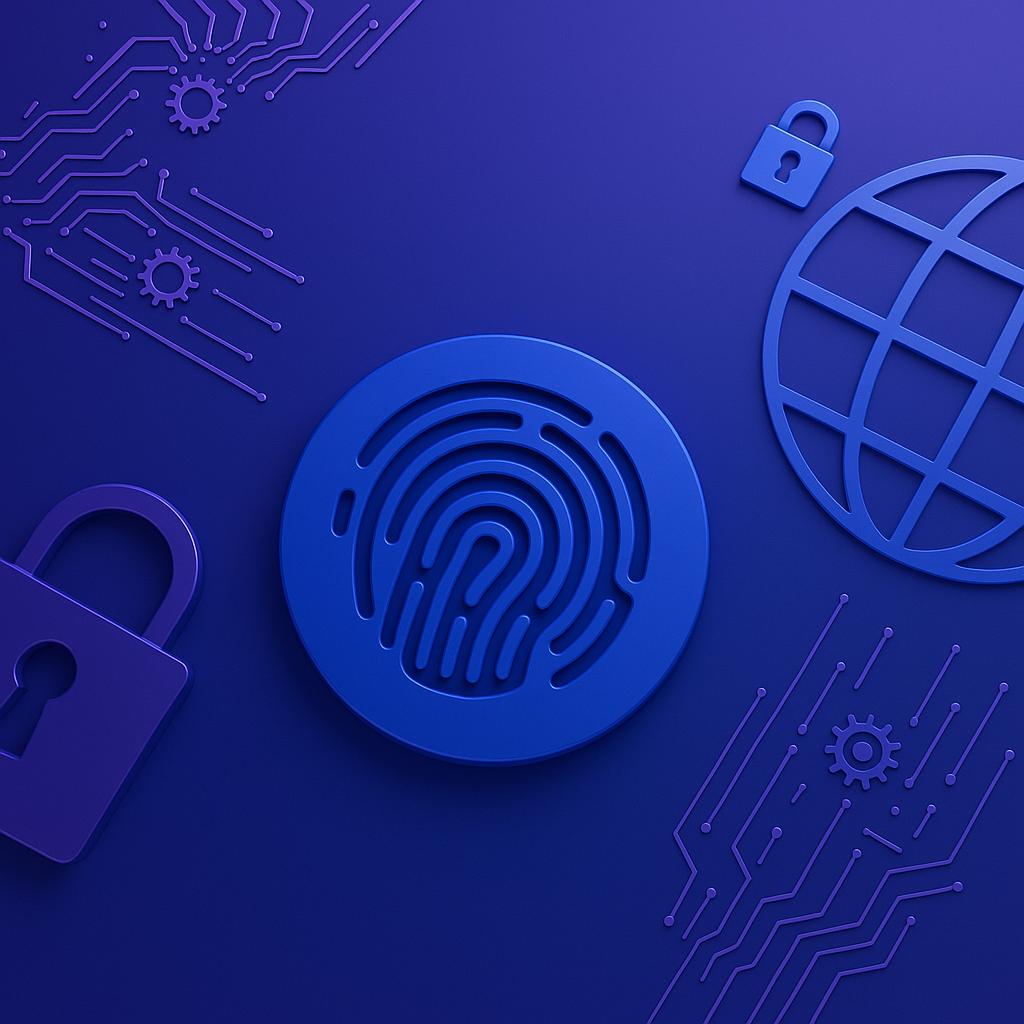
Governance, Compliance, and Risk Management for Healthcare AI Agents
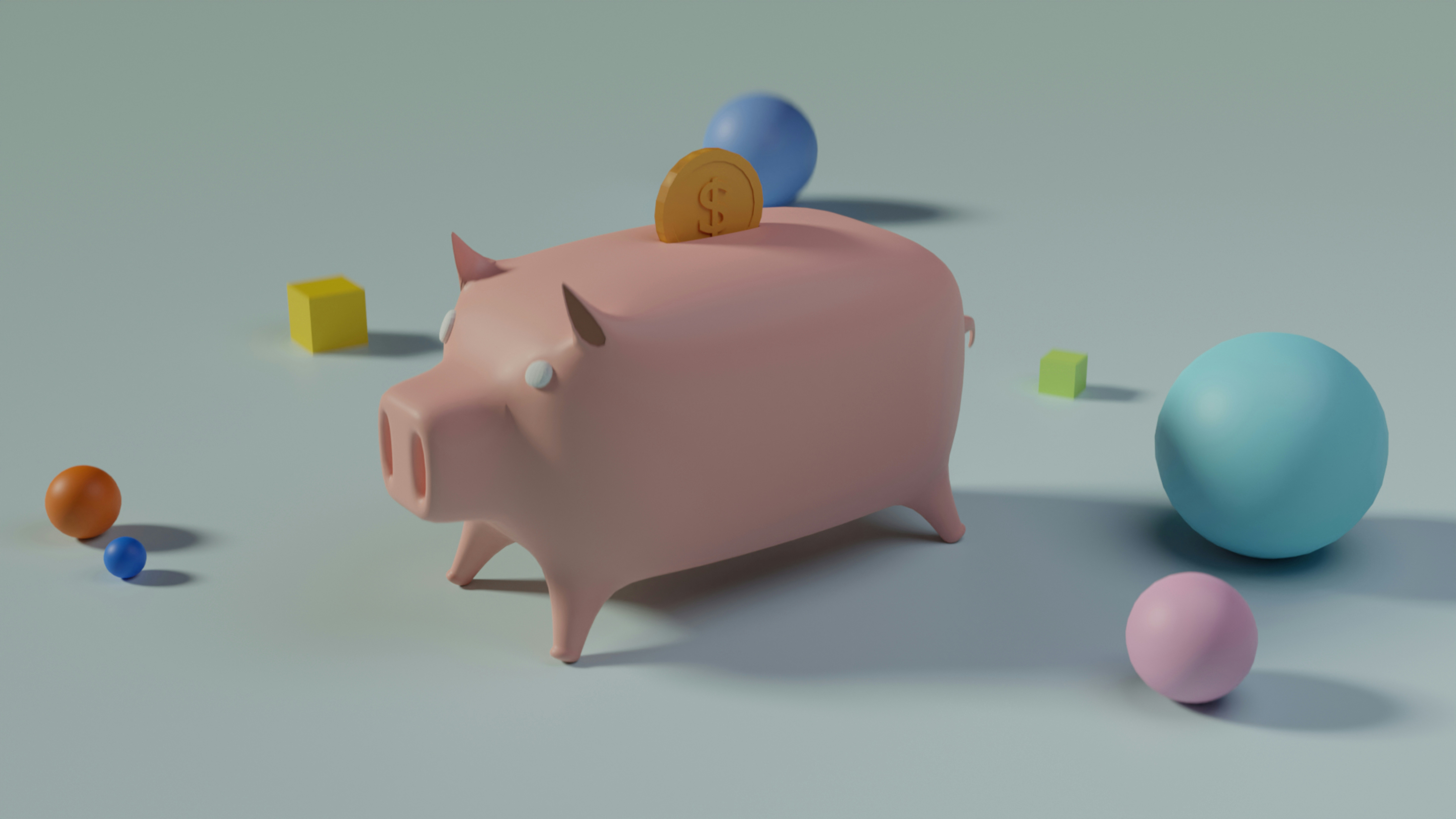
How AI is Helping Payers Recover Millions in Denied Claims
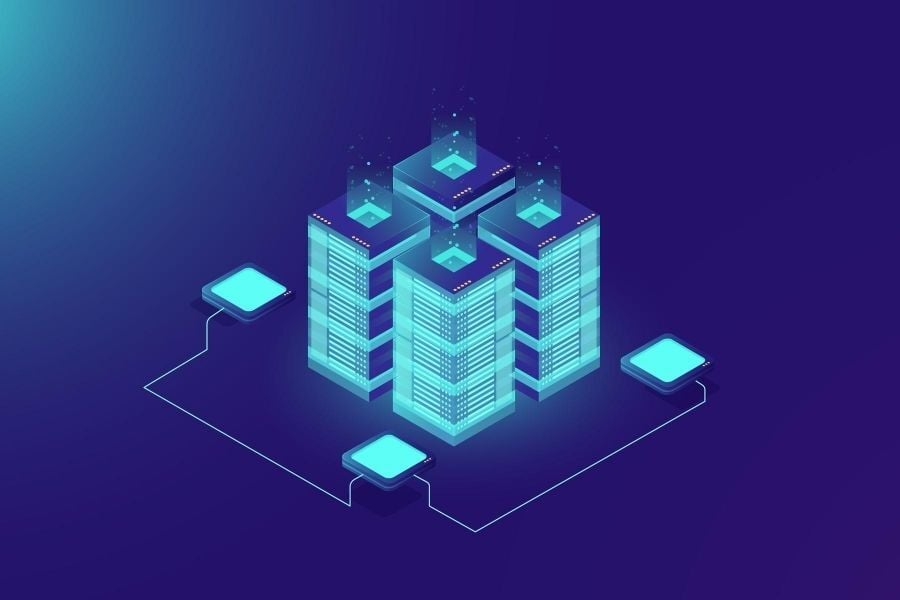